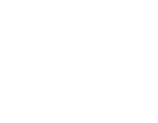
(SEML)
(S-Expression Markup Language)
No such thing as too many parentheses.
Download SEMLSEML is a markup language that compiles to clean, well-formated HTML.
(;; This is a (sexy) comment)
(section#home.awesome
((h1 "This rocks !")
(p.center "What a wonderful language, isn't it ?")))
<!-- This is a (sexy) comment -->
<section id="home" class="awesome">
<h1>This rocks !</h1>
<p class="center">
What a wonderful language, isn't it ?
</p>
</section>
SEML is a powerful markup language based on Lisp-like structures, that compiles to standardized, clean and well-formatted HTML5 (and CSS3 too, soon).
SEML purely embodies the power of S-Expressions. Everything you manipulate in SEML is some kind of S-Expression, just like in Lisp and its descendants.
Indentation-sensible languages are cool, but sometimes you just need the flexibility of good old structures. In SEML, you choose to indent your code the way you like it. Whitespaces, tabs, or spaghetti? Whatever rows your boat.
Templating can be a repetitive task; that's why SEML includes syntactic sugar for common idioms to speed up your development time. You haven't anything new to learn, though: each one of them is a valid SEML S-Expression structure.
SEML is logic-less, which means you can't embed external, dynamic content inside your views. This is a choice: by not being able to embed scripts, you can purely focus on the template -- and nothing else.
SEML goes far beyond simple, classical logic-less languages: you can't embed JavaScript nor stylesheets directly in your templates. Behind such seemingly horrid rules lies in fact a simple, well-known (but alas not always respected) concept: don't mix up everything !
S-Expressions are quick and easy to parse, which means SEML can deliver your outputs flawlessly, in the glimpse of an eye.
Both installation methods assume you have an operational Ruby (>= 1.9) environment available.
Current version: 0.0.1
Recommended installation. You'll need the command-line application gem
.
Run gem install seml
in your command line.
And... You're done!
Don't forget to run gem update seml
once in a while to stay up-to-date !
Custom installation. You'll need the command-line application git
.
Run git clone https://github.com/loganbraga/seml
in your command line, and cd seml
.
You now have a working git repository containing the source code of SEML. You should know what you want to do with it from now, right...?
Oh, and don't forget to run git pull
inside the directory once in a while.
In this part, we assume you have already downloaded and/or installed SEML and can therefore use the command-line application seml
.
SEML draws its power from S-Expression and Lisp-like structure. As such, you will obviously feel instantly at home if you already have experience with similar languages, but it is not a requirement. Everyone is able to grasp the power of S-Expressions after a little bit of practice !
Now sit back, relax, and enjoy this quick ride that will get you started on using SEML in your everyday templating tasks...
Hint: You can click on every title to collapse the corresponding section, or you can collapse everything at once.
Before we really dive in, we must agree on something: every example you will see below will have been compiled by the command-line application before showing the HTML result.
But we won't write that everytime. That would be silly.
So, now, you're in: each time you'll see SEML and HTML codes side by side, you'll just know there was a compilation in-between, even if that's not written. You smart boy.
Wait, now you may be wondering how that compilation was done. It's rather simple, in fact:
seml myFile.seml myOutput.html
SEML follows POSIX conventions for command-line apps. Those three line above are equivalent.
seml -i myFile.seml -o myOutput.html
seml --input=myFile.seml --output=myOutput.html
By default, your first argument is the input file (which can also be explicitly stated with -i or --input), and the second the output file (same with -o or --output).
If there's no output file, SEML will send its result to the standard terminal output. If there's no input, it will take it from standard terminal input.
Understanding the basics of S-Expressions is essential in order to fully master SEML.
In traditional Lisp and in SEML, an atom is a part that can't be divided into something smaller on its own. That is, all of these things are valid atoms: foo
, +
, 132323
, z
and *abc123*
.
On the other hand, 4 ab
is not an atom (it is an insignificant group of two atoms in this context), and neither is (+ 4 8)
, because it is a list of three atoms. Which leads us to S-Expressions.
An S-Expression is, as wonderfully put by Wikipedia, either an atom or a group of two S-Expressions wrapped in parentheses. That is, (+ 1 4)
, (foo bar baz)
, abc
, (3 abc (zz y))
and ()
are all valid S-Expressions.
Similarly, (4 5 (8)
and f (gh)
are ill-formed S-Expressions.
Writing templates in SEML is pretty straight-forward; all you have to do is enclose your tags, which are atoms, inside parenthesis and follow the simple Sexps rules above. Piece of cake!
Parameters of the tags are what will be rendered inside them. They can be written after one or more whitespace after the tag atom. If a parameter is an atom, it can be written as is. If it is an S-Expression, it must be enclosed in parentheses. If there's more than one parameter, the whole group must be enclosed in parentheses too.
For example, try to understand this little something:
(h1 "This is a title !")SEML
(hr)
(p "And this a paragraph.")
<h1>This is a title</h1>HTML
<hr>
<p>
And this a paragraph.
</p>
See how you don't need to put parentheses around the arguments for h1
and p
? That's because the argument is an atom (here, a string).
In this next example, we use nested arguments. That is, S-Expressions arguments. Doesn't that sound exciting?
(sectionSEML
(div
(h3 "Oi y'all!")
(p
("Woaw, a paragraph!"
br
"Still inside!"))))
<section>HTML
<div>
<h3>Oi y'all!</h3>
<p>
Woaw, a paragraph.
<br>
Still inside!
</p>
</div>
</section>
Pretty neat, if you ask me.
Adding attributes in SEML is fairly easy. You just have to use an exclamation mark next to the tag, followed by an equal sign and the value of the attribute. This results in something like this :
(img!src="foo.png"!title="Bar")SEML
<img src="foo.png" title="Bar">HTML
Because classes and IDs are so common, SEML provides a way to write them more comfortably. The syntax in inspired from CSS syntax, and Haml.
You simply use CSS selectors: #myID
for IDs and .myClass
for classes.
(div#menu.center.collapse)SEML
<div id="menu" class="center collapse">HTML
</div>
You can obviously combine them all the way you want, in any order. Classes can be cumulated; multiple or non-unique IDs are semantically incorrect, but won't raise any error.
Special HTML characters are automatically escaped into valid HTML entities in SEML. No worries!
éôü&><SEML
é
éôü&><HTML
&eacute;
Don't start escaping them yourself if you're not sure you should do it or bad things will happen, as seen in the second line.
In SEML, there are two types of comments.
SEML comments are used only for the sake of your SEML template; they will not be rendered in the final output.
You write them using the Lisp-like comment notation ;; Comment
(two semicolons).
;; This is a SEML commentSEML
;; it won't be rendered
HTML comments are comments that will be rendered in the final output.
You write them using the Lisp-like comment notation ;; Comment
(two semicolons) inside a list.
(;; This is an HTML comment)SEML
(;; it will be rendered)
<!-- This is an HTML comment -->HTML
<!-- it will be rendered -->
SEML comes bundled with a lot of syntactic sugar for frequently used idioms.
A syntactic sugar is called by using the star-variable notation, popular (well, not so) amongst Lispers. For example, you can do this:
(*doctype*)SEML
<! DOCTYPE HTML>HTML
Or, similarly, this:
(*utf8*)SEML
<meta charset="utf-8">HTML
You can get an exhaustive list of all syntactic sugars in SEML in the documentation.
From now on, you should be able to write and compile most of your everyday templating tasks in SEML. Awesome! Pat yourself on the back.
There's still though a lot you can do to fully embrace SEML or help with the project.
To become a SEML expert or simply find some more informations, you can check the comprehensive documentation. If it doesn't solve your problem, you might try to contact us.
There's no better way to learn something than diving right into its core. If you're interested, take a peek at the source code. You may even want to contribute something!
We accept and value every support we can get. If you like SEML, talk about it! A big community is something we can all benefit from.